- How to use Arrays in Arduino coding?
- How to save Arduino Serial data in TXT, CSV and Excel file without using data logger shield?
- What to do if the theme editor option in WordPress dashboard disappeared?
- How analog pin works & how PWM signal is obtained in Arduino UNO/Nano?
The use of data logger shield in Arduino is rather critical and everyone may not have the data logger shield readily available to create the log of readings in Arduino.
So this article explains how to save Arduino Serial data in TXT, CSV and Excel file without using data logger shield?
Also refer our Arduino Tips & Tricks and Arduino Tested Projects. Download all Library Files and Fritzing Parts file for better study with Arduino.
Now suppose we have create log of real time temperature and humidity, using DHT11 sensor with Arduino. For that we will use a simple code, as follows.
Simple Arduino code
To understand the process of saving Arduino Serial data in txt, csv and Excel file, we will use a simple Arduino code which uses Serial.print() commands. Let us see the code first.
/* * Code for the article: * How to save Arduino Serial data * in TXT, CSV and Excel file * without using data logger shield? * Vidyasagar Academy, Akola * www.vsa.edu.in */ #include <dht.h> int output=7; // connect output pin of DHT11 to pin-7 int readdata; // DHT data to store int Temperature; // variable to store temperature value int Humidity; // variable to store humidity value dht DHT; // creating DHT object void setup() { Serial.begin(9600); } void loop() { readdata = DHT.read11(output); Temperature = DHT.temperature; // reading temperature Humidity = DHT.humidity; // reading humidity Serial.print(Temperature); Serial.print(","); Serial.println(Humidity); delay(1000); // create reading log at every second }
Note: To run this code in Arduino IDE you will need DHT library. You can download it from this link. To add the downloaded zipped file, open Arduino IDE and click on “Sketch” menu. Then go to “Include Library” and then click on “Add .Zip Library, as shown below.
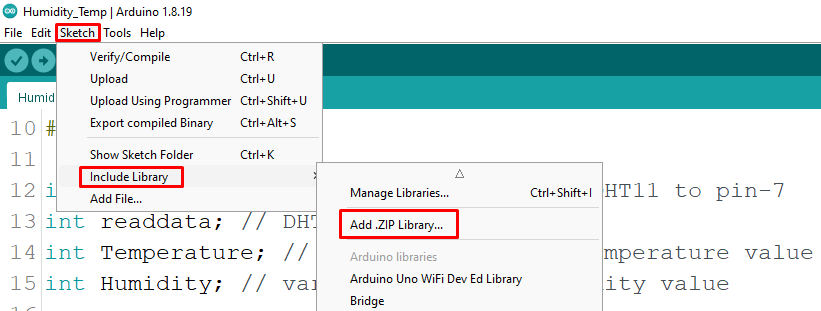
It is important to note the following piece of code used to print the Serial data. This is because we have to import the Serial data with comma delimited values in Excel file.
Serial.print(Temperature);
Serial.print(",");
Serial.println(Humidity);
The first line in above code prints the “Temperature” value and the second line puts a comma (,) after the Temperature value.
Then the third line prints “Humidity” value. Since we used Serial.println() in this third line, due to carriage return, the next two values will be printed in next line and so on…
Download Data logging software
Now that you are ready with the code, follow these steps for data logging –
- Download CoolTerm data logger software from this link (File size: 20MB).
- After downloading, unzip the file and copy the folder inside the zip file on your PC.
- Open the folder and right click on CoolTerm.exe icon and create desktop shortcut on your PC.

- Now connect your Arduino board through data cable to your PC and upload the code in Arduino UNO or Arduino nano.
- Do not open Serial Monitor or Serial Plotter. Just note down the COM port to which your Arduino is connected to. In my case, it is connected to COM3 port.
- Now open CoolTerm application.
- Click on “Options” menu and then click on “Port”. This field should show you the COM port number to which your Arduino is connected to. Then click OK. Here I am using the Baudrate as 9600.
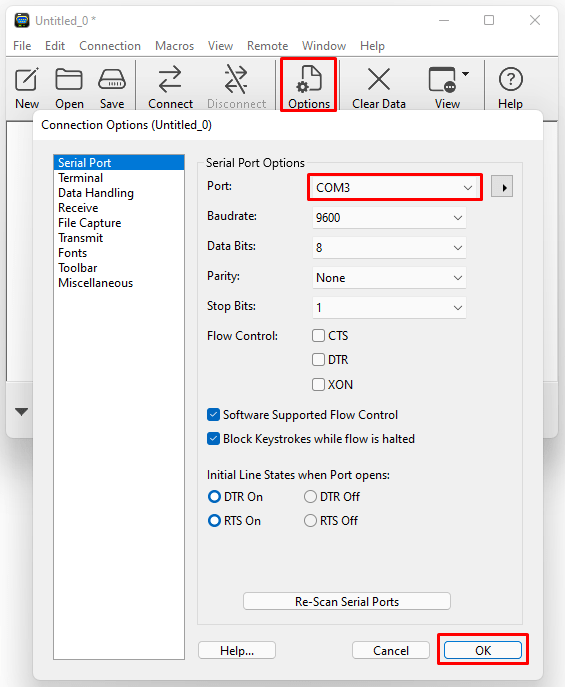
- Click on “Connections” in menu, go to “Capture to Text/Binary File” and click on “Start”, as shown below.
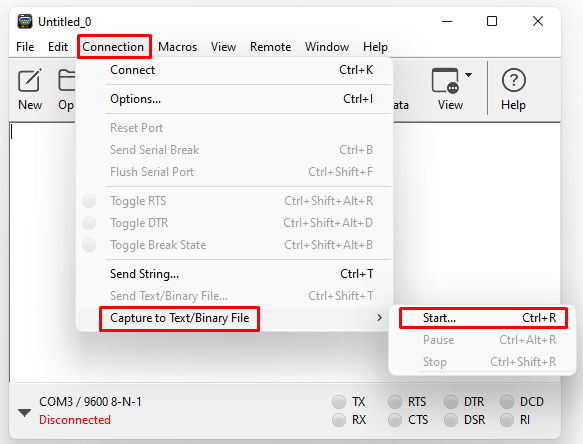
- Now a new window will open where you have write the name of the file.
- Finally click on “Connect” button of CoolTerm application to start recording the data entries in the text file, as shown below.
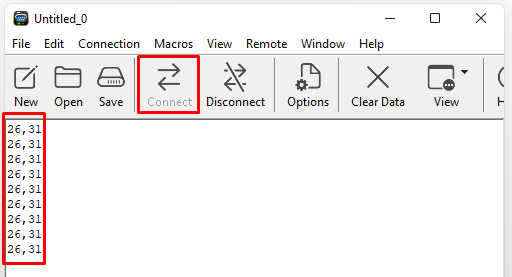
- Once you have sufficient data entries, click on “Connections”, go to “Capture to Text/Binary File” and click on “Stop”.
- Then click on “Disconnect” button.
- This will complete data logging process.
Import TXT file in Excel
- You must have the txt file, generated by CoolTerm either on desktop or in a folder.
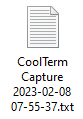
- Now open Microsoft Excel and click “Open” in its menu. Then click on “All files” in bottom right corner as shown below and select “Text Files (*.prn, *.txt, *.csv)”.
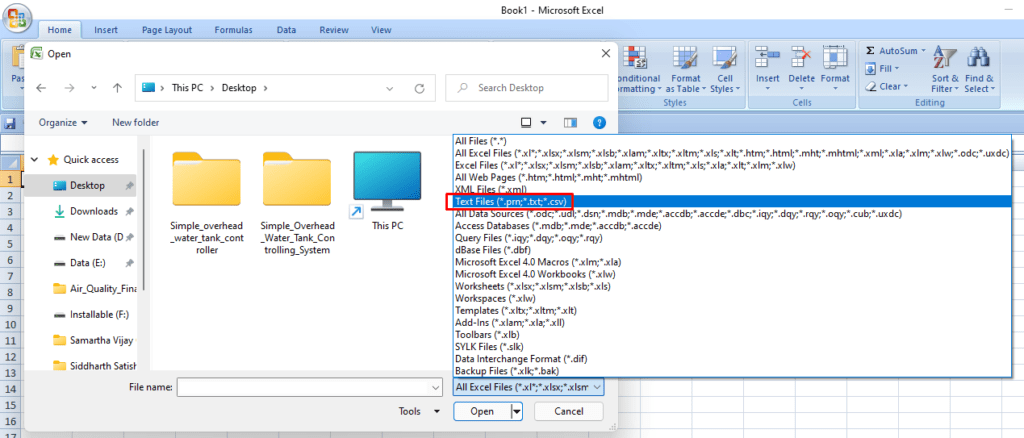
- Now it will show you your text file generated by CoolTerm. Select the file and click “Open”.
- This will open text import wizard.
- Select “Delimited” button and then click “Next”.
- Now as shown below, deselect “Tab” option and select “Comma” option, then click “Next”.
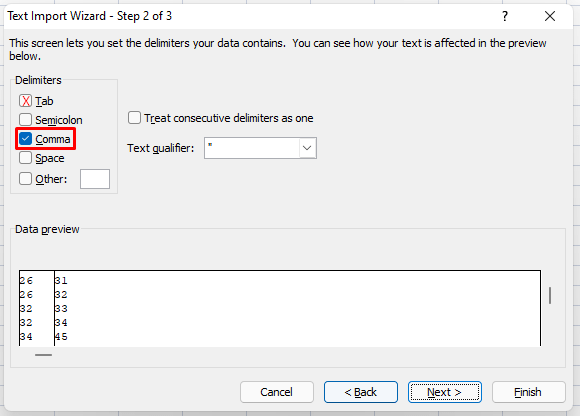
- Finally click “Finish” and now your TXT file will be converted into two columns in Microsoft Excel table, as shown below.
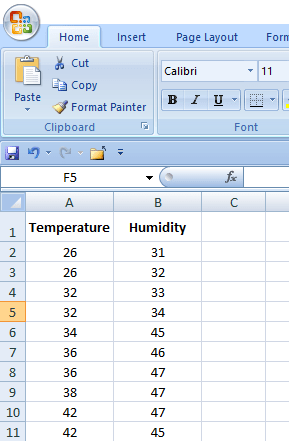
How to create graph of the data entries?
- Since your data entries are imported into MS Excel in two columns, you can now create graph of the entries.
- Insert one row above the data entries and give headings to the two columns as Temperature and Humidity, as shown below.
- For that click on “Insert” menu and then select “Line” graph option.
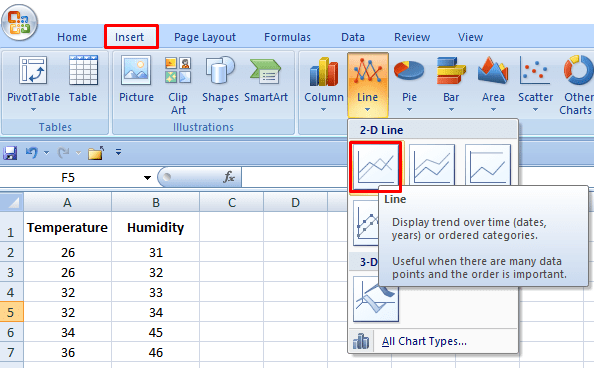
- Instantly the graph will be displayed as follows.
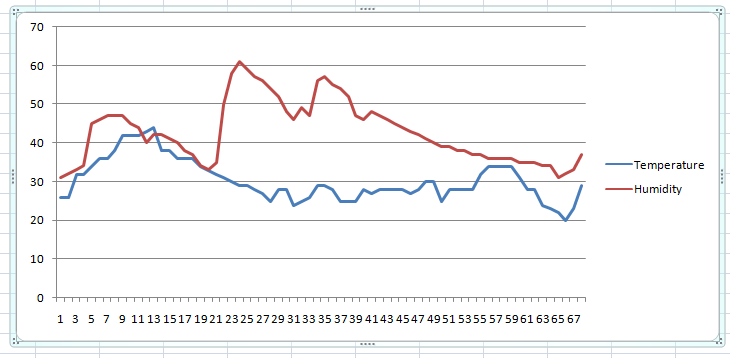
Still More simple solution…
Just ignore all above methods and let us see the simplest possible method, as given below.
- Run the code of Temperature and Humidity sensor, given above.
- Then open the Serial Monitor in Arduino IDE.
- You will see the readings like this –
27, 22
27, 22
28, 20
27, 23
29, 20
30, 21
29, 20
28, 20
28, 21
29, 20
29, 20
- Now copy these readings from Serial Monitor and paste them in a filename.txt file.
- Then import this .txt file in MS Excel.
- Create a header row and give labels to the two quantities like Temperature & Humidity.
- The click on “Data” tab in MS Excel and import your .txt file with “Comma delimited” style, as shown below.
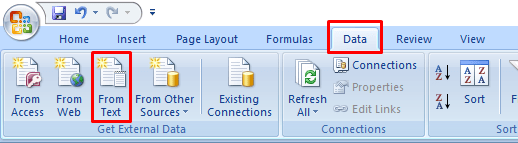
- Then click on “Insert” tab in MS Excel and create graph.
That’s it…! You did it…!