Click here to register for summer batches of robotics. Click here for details of courses.
- AR-AD1: Sanguinoscope Blood Group Typing Project
- AR-AD2: Arduino Advanced Calculator
- AR-AD3: Arduino based Fastest Figure First Controlling System for Quiz Competition
- Convert Text into Morse Code with beeps & back into Text with Arduino
What is Morse Code?
Morse code is a method of communication that uses short and long signals, represented by dots and dashes, to represent letters, numbers, and punctuation.
It was invented in the 1830s and 1840s by Samuel Morse to be used with the telegraph, an early electrical communication system.
Let us understand details of Morse code:
Signals: Morse code uses two basic signal lengths: dots (short) and dashes (long).
Encoding: These dots and dashes are combined in specific sequences to represent letters, numbers, and punctuation.
Transmission: Morse code can be transmitted in a variety of ways, including electrical pulses, sound beeps, or light flashes. While less common today, Morse code was once a vital tool for long-distance communication. It played a significant role in the early days of the telegraph and even had applications in maritime communication and early radio.
Morse Code Reference Chart
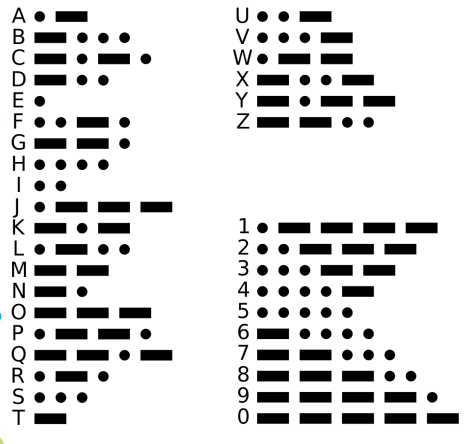
How to construct the project?
For that you need following material -
- Arduino UNO/ Arduino Nano
- Data cable
- Buzzer
- Jumper Wires
Make sure that Arduino IDEWhat is Arduino IDE? Arduino IDE stands for Integrated Development Environment. It is also called as Arduino software in general language or Arduino compiler. It is used to convert high level programming language like C/C++ program into machine code i.e. into low level language. Software is installed on your PC. If not you can download it and install.
Now let us see the code and the connection details to construct the circuit.